Angular API Integration
Integrating APIs into Angular applications is a crucial aspect of modern web development, enabling seamless communication between client-side apps and external services. This article delves into the fundamentals of Angular API integration, exploring how to efficiently fetch, handle, and display data. Whether you're a seasoned developer or new to Angular, mastering these techniques will enhance your ability to build dynamic and interactive applications.
Introduction to Angular API Integration
Angular, a powerful front-end framework developed by Google, is widely used for building dynamic web applications. One of its key features is the ability to seamlessly integrate with RESTful APIs, enabling developers to fetch, manipulate, and display data efficiently. This integration is crucial for creating interactive and responsive user interfaces that communicate with back-end services in real-time.
- Angular's HttpClient module simplifies HTTP requests and responses.
- Services in Angular facilitate code reusability and separation of concerns.
- Observables and RxJS provide powerful tools for handling asynchronous operations.
By leveraging Angular's robust API integration capabilities, developers can build applications that not only perform well but also offer a rich user experience. The framework's modular architecture, combined with TypeScript's strong typing, ensures that applications are scalable and maintainable. Whether you're developing a simple application or a complex enterprise solution, understanding how to effectively integrate APIs in Angular is essential for delivering high-quality software.
Setting up the Development Environment
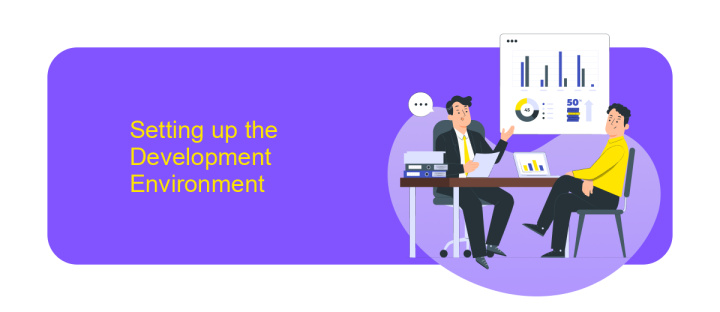
To begin setting up your development environment for Angular API integration, you need to have Node.js and npm installed on your system. These tools are essential as they provide the necessary runtime and package management capabilities for Angular applications. You can download and install the latest version of Node.js from its official website, which will also install npm automatically. Once installed, verify the installation by running node -v
and npm -v
in your terminal to check their respective versions.
After setting up Node.js and npm, proceed to install the Angular CLI, a command-line interface that simplifies Angular development. Run the command npm install -g @angular/cli
to install it globally on your system. With Angular CLI installed, you can create a new Angular project by using ng new project-name
. If your project involves API integrations, consider using ApiX-Drive, a platform that facilitates seamless data exchange between various applications. ApiX-Drive can help streamline your integration processes, offering a user-friendly interface to connect different APIs without extensive coding.
Making API Requests with HttpClient
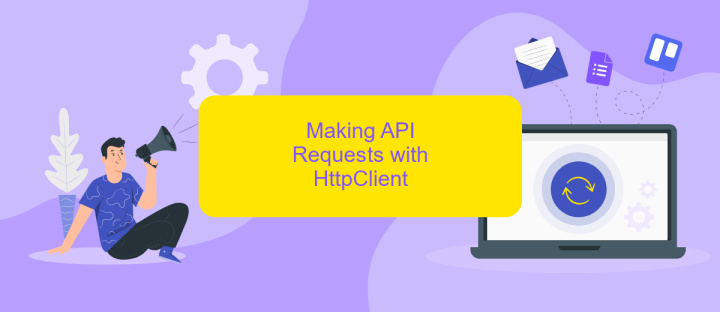
Integrating APIs into your Angular application is streamlined with the HttpClient module, which simplifies the process of sending HTTP requests and handling responses. To begin, ensure that HttpClientModule is imported into your app module. This will allow you to inject HttpClient into your services or components, enabling seamless communication with external APIs.
- First, import the HttpClientModule in your app.module.ts file and add it to the imports array.
- Inject HttpClient into your service or component constructor where you plan to make API calls.
- Use HttpClient methods such as get(), post(), put(), or delete() to interact with your API. These methods return an Observable, so you can subscribe to them to handle the data or errors returned by the server.
By leveraging HttpClient, developers can efficiently manage API requests and responses, ensuring a robust integration within Angular applications. Its built-in features, such as interceptors and error handling, provide additional layers of functionality, making it a powerful tool for developers aiming to create dynamic, data-driven applications.
Handling API Responses and Errors
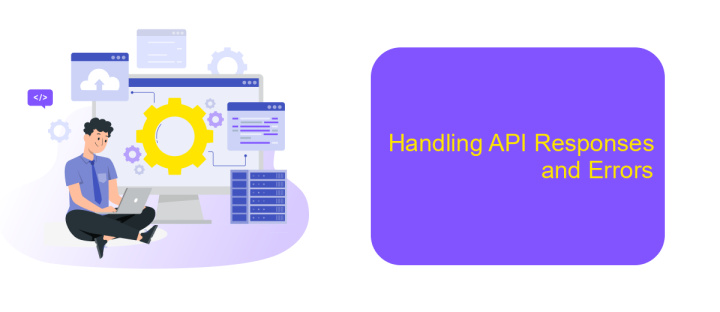
When integrating APIs in Angular, effectively handling responses and errors is crucial for a seamless user experience. Once an API call is made, the application should be prepared to process both successful and unsuccessful responses. This involves parsing the returned data and updating the application's state accordingly. Angular's HttpClient module provides a streamlined way to manage these responses using observables, allowing developers to subscribe to the data stream and react to changes.
Error handling is equally important, as it ensures the application can gracefully manage unexpected situations. By implementing error handling logic, developers can provide users with meaningful feedback and maintain application stability. Angular's HttpClient offers built-in methods to catch errors, enabling developers to define custom error-handling strategies.
- Use the
catchError
operator to handle errors in HTTP requests. - Display user-friendly error messages to inform users of issues.
- Log errors for further analysis and debugging.
- Implement retry logic for transient errors to improve resilience.
By carefully managing API responses and errors, developers can enhance the reliability and user experience of their Angular applications. A thoughtful approach to error handling not only improves application robustness but also builds user trust by demonstrating a commitment to quality and reliability.

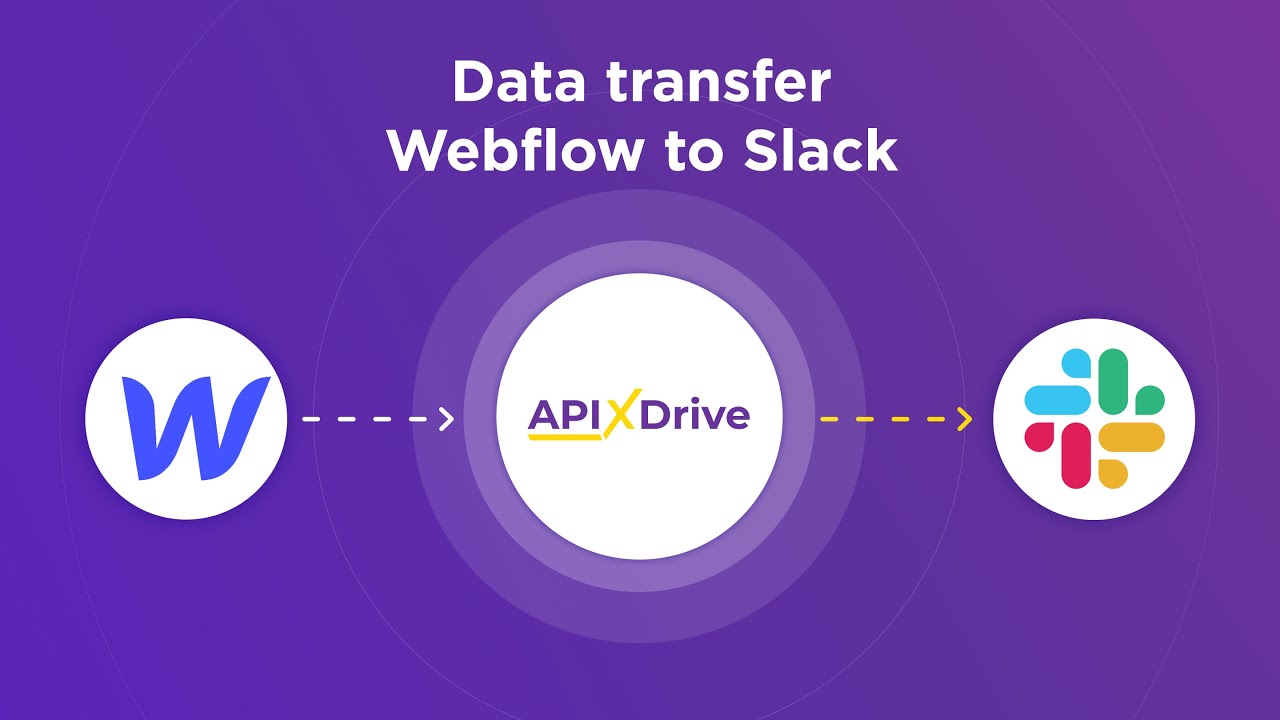
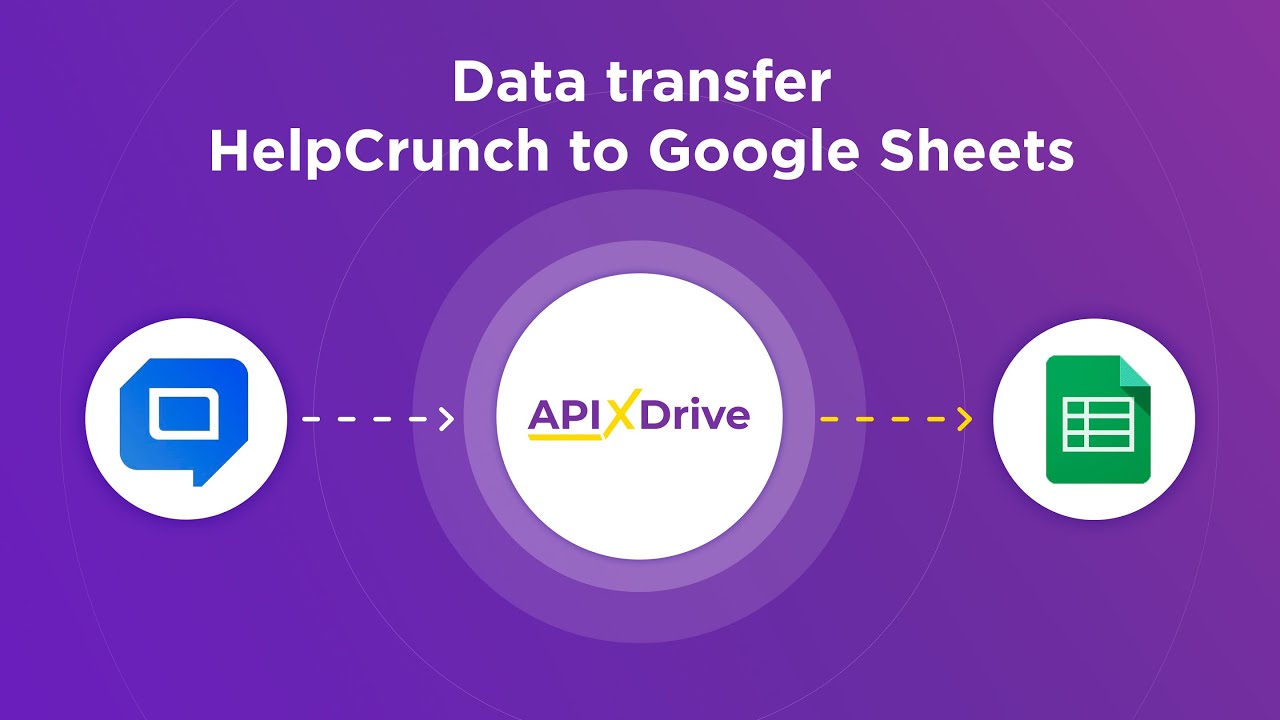
Best Practices and Advanced Techniques
When integrating APIs in Angular applications, one best practice is to leverage Angular's HttpClient module. This module simplifies HTTP requests and error handling, ensuring a seamless data exchange process. Utilize interceptors to manage headers, authentication tokens, and logging, which enhances security and debugging capabilities. Additionally, implement RxJS operators to handle asynchronous data streams efficiently, allowing for more responsive and scalable applications.
For advanced techniques, consider using ApiX-Drive to streamline API integrations. This service provides a user-friendly interface to connect various APIs without extensive coding, saving time and reducing errors. It supports automation, enabling real-time data synchronization across different platforms. Moreover, ensure that your application architecture follows the SOLID principles, promoting maintainability and scalability. Regularly test your integrations with tools like Postman to validate endpoints and responses, ensuring robust and reliable API interactions.
FAQ
How do I integrate a third-party API in an Angular application?
What are the best practices for handling API keys in an Angular app?
How can I handle errors when integrating APIs in Angular?
What is the role of services in Angular API integration?
How can I automate and configure API integrations without writing extensive code?
Time is the most valuable resource for business today. Almost half of it is wasted on routine tasks. Your employees are constantly forced to perform monotonous tasks that are difficult to classify as important and specialized. You can leave everything as it is by hiring additional employees, or you can automate most of the business processes using the ApiX-Drive online connector to get rid of unnecessary time and money expenses once and for all. The choice is yours!