Python API Integration
Integrating Python APIs has become an essential skill for developers seeking to enhance application functionality and streamline workflows. By leveraging Python's robust libraries and tools, developers can easily connect diverse systems, automate tasks, and retrieve or manipulate data efficiently. This article explores the fundamentals of Python API integration, providing insights and practical examples to help you seamlessly incorporate APIs into your projects, unlocking new possibilities and improving overall performance.
Understanding APIs and Their Role in Python Integration
Application Programming Interfaces (APIs) are crucial in modern software development, acting as bridges that enable different software systems to communicate with each other. In the context of Python, APIs allow developers to integrate Python applications with external services, libraries, or platforms. This integration is essential for building scalable and efficient applications, as it enables Python programs to utilize features and data from other services without reinventing the wheel.
- Ease of Integration: APIs provide a standardized way to access external services, simplifying the integration process.
- Reusability: By using APIs, developers can leverage existing functionalities, reducing development time and effort.
- Scalability: APIs allow applications to scale by integrating with cloud services and other scalable platforms.
- Interoperability: APIs enable Python applications to interact with software written in different languages, enhancing versatility.
In Python, working with APIs is facilitated by various libraries and frameworks, such as Requests and Flask, which streamline the process of sending and receiving data over the internet. Understanding how to effectively use APIs is a vital skill for Python developers, as it opens up a world of possibilities for creating dynamic and interactive applications that can seamlessly interact with other digital services.
Setting Up Your Python Environment for API Interaction
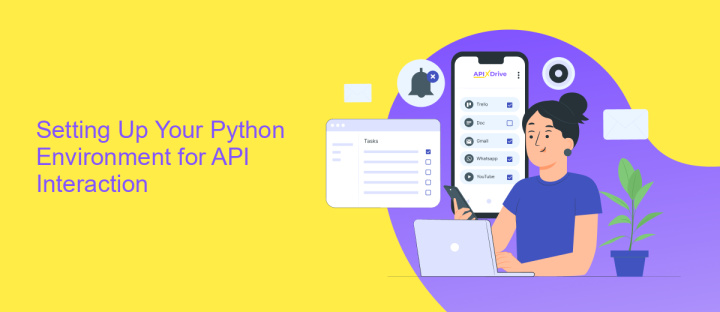
To effectively interact with APIs using Python, it's essential to set up a robust environment. Start by ensuring you have Python installed on your system. You can download the latest version from the official Python website. Once installed, use a virtual environment to manage your dependencies. This can be done by executing python -m venv myenv
in your terminal, where "myenv" is the name of your virtual environment. Activate it using source myenv/bin/activate
on macOS/Linux or myenv\Scripts\activate
on Windows. This setup helps prevent conflicts between different projects.
Next, install essential libraries such as requests
for handling HTTP requests and json
for parsing JSON data. You can do this by running pip install requests
. For more complex integrations, consider using services like ApiX-Drive, which simplifies the process of connecting various applications and automating workflows without requiring extensive coding knowledge. By configuring your environment correctly and leveraging powerful tools, you'll be well-equipped to handle API interactions smoothly and efficiently.
Making API Requests with Python Libraries (e.g., Requests, urllib)
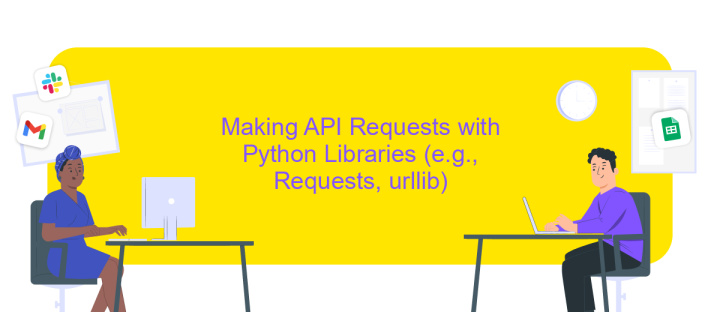
Python provides several libraries to facilitate API requests, making it easier for developers to interact with web services. Among the most popular are the Requests library and urllib. These tools allow you to send HTTP requests and handle responses efficiently. Whether you're fetching data from a RESTful API or submitting data to a web service, these libraries offer a straightforward approach to managing HTTP methods like GET, POST, PUT, and DELETE.
- Requests: This library simplifies HTTP requests in Python. You can install it using pip and start sending requests with minimal code. It handles various complexities such as authentication, sessions, and cookies.
- Urllib: A module included in the Python standard library, urllib provides functionalities to work with URLs and handle HTTP requests. While powerful, it requires more boilerplate code compared to Requests.
Choosing between Requests and urllib depends on your specific needs. Requests is favored for its simplicity and ease of use, while urllib is a good choice if you prefer using a built-in library without external dependencies. Both options are excellent for making API requests, ensuring that Python remains a versatile tool for web integration tasks.
Handling API Responses and Data Processing
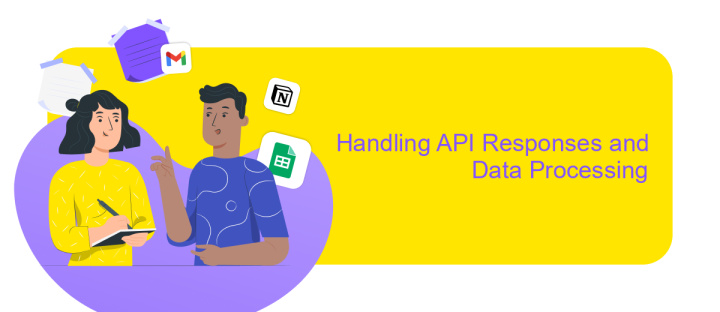
When working with Python API integration, effectively handling API responses is crucial. Upon receiving a response, the first step is to check the status code to ensure the request was successful. A status code of 200 indicates success, while codes like 404 or 500 signify errors that need addressing. Once the response is verified, the next task is to parse the data.
Most APIs return data in JSON format, which can be easily handled in Python using the built-in `json` module. Convert the JSON response into a Python dictionary for seamless data manipulation. This allows you to access and process the data efficiently, tailoring it to your application’s requirements.
- Check the response status code.
- Parse JSON data into a Python dictionary.
- Handle exceptions for error codes.
- Extract necessary information from the data.
After processing the data, it's essential to implement error handling mechanisms. This ensures that your application can gracefully manage unexpected responses or data anomalies. By structuring your code with appropriate exception handling, you enhance the robustness and reliability of your API integration, providing a seamless experience for end-users.
Best Practices and Common Pitfalls in Python API Integration
When integrating APIs with Python, it's crucial to adhere to best practices to ensure seamless functionality and maintainability. Start by thoroughly reading the API documentation to understand its capabilities and limitations. Use a reliable HTTP library like `requests` to handle HTTP requests efficiently. Implement error handling to manage exceptions gracefully and log errors for debugging. Secure your API keys by storing them in environment variables or configuration files, and never hardcode them into your scripts. Additionally, consider using a service like ApiX-Drive to automate data integration processes, which can simplify the management of multiple APIs.
Common pitfalls in Python API integration include ignoring rate limits, which can lead to throttling or blocking of your API access. To avoid this, implement mechanisms to respect these limits, such as using exponential backoff strategies. Another frequent mistake is neglecting data validation, which can result in corrupted or unexpected data. Always validate incoming data and sanitize outputs to maintain data integrity. Lastly, keep your dependencies up to date to avoid security vulnerabilities and ensure compatibility with the latest API versions. By following these practices, you can enhance the reliability and efficiency of your Python API integrations.
FAQ
What is an API and how is it used in Python?
How do I authenticate when using a Python API?
What are some common challenges in Python API integration?
How can I automate API integration tasks in Python?
What is the best way to test a Python API integration?
Time is the most valuable resource in today's business realities. By eliminating the routine from work processes, you will get more opportunities to implement the most daring plans and ideas. Choose – you can continue to waste time, money and nerves on inefficient solutions, or you can use ApiX-Drive, automating work processes and achieving results with minimal investment of money, effort and human resources.