Integration Test Spring Boot REST API
Integration testing is a crucial step in ensuring the reliability and functionality of a Spring Boot REST API. By simulating real-world scenarios, these tests validate the interaction between different components of the application. This article delves into effective strategies for conducting integration tests in a Spring Boot environment, highlighting best practices and tools to streamline the process, ultimately enhancing the robustness and performance of your RESTful services.
Introduction to Integration Testing and Spring Boot REST APIs
Integration testing plays a crucial role in ensuring that different components of an application work together as expected. It sits between unit testing and end-to-end testing, focusing on the interaction between integrated units. For Spring Boot REST APIs, integration tests validate the collaboration between controllers, services, and repositories, ensuring that HTTP requests and responses function correctly across the application layers.
- Verify the API endpoints' behavior with real data and dependencies.
- Ensure the compatibility of multiple components within the application.
- Detect issues that may not be obvious during unit testing.
- Facilitate regression testing by validating the entire workflow.
Spring Boot simplifies integration testing by providing tools and annotations that streamline the setup of test environments. Its embedded server capabilities enable developers to test REST APIs without deploying them to an external server, making the process efficient and less time-consuming. By leveraging Spring's testing framework, developers can execute comprehensive integration tests, thereby enhancing the reliability and robustness of their REST APIs. This approach not only improves code quality but also boosts confidence in the application’s performance in production environments.
Setting up the Test Environment
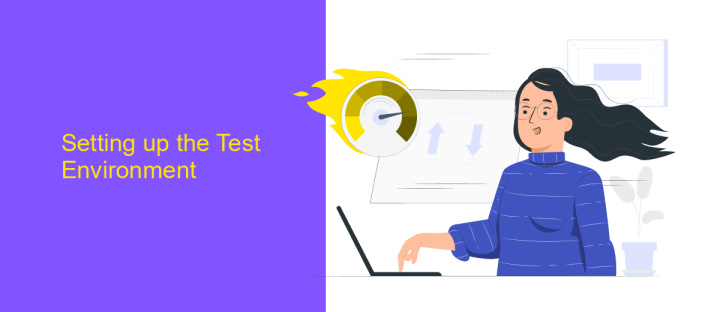
To set up the test environment for your Spring Boot REST API integration tests, begin by configuring a dedicated testing database. This ensures that your tests do not interfere with your production data. Utilize an in-memory database like H2, which is ideal for testing purposes due to its lightweight nature and ease of setup. Configure your application to use this database profile during test execution by specifying the relevant properties in your `application-test.properties` file.
Next, incorporate a build tool like Maven or Gradle to manage your project dependencies efficiently. Ensure that your `pom.xml` or `build.gradle` file includes dependencies for JUnit, Spring Boot Test, and any additional libraries necessary for your specific testing requirements. If your application involves external integrations, consider using ApiX-Drive to simulate and manage these integrations seamlessly. This tool can help automate data flows and test API interactions, ensuring your integrations are functioning correctly without manual intervention. Finally, set up a continuous integration (CI) pipeline to automate your testing process, ensuring that your tests run consistently with each code change.
Writing Integration Tests with Spring Test
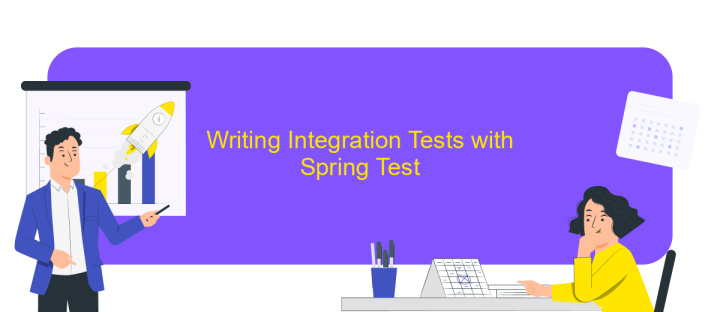
Integration testing in Spring Boot REST API ensures that different parts of the application work together as expected. Spring Test provides a comprehensive framework to create integration tests that interact with the application context, making it ideal for testing REST APIs. By using Spring Test, developers can simulate HTTP requests and verify the responses, ensuring the API behaves correctly under various scenarios.
- Set up your test class with
@SpringBootTest
to load the full application context. - Use
@AutoConfigureMockMvc
to configure MockMvc, allowing you to perform HTTP requests. - Inject
MockMvc
into your test class to simulate API calls and assert responses. - Write test methods annotated with
@Test
, using MockMvc to perform requests to your REST endpoints. - Verify the HTTP status, response content, and other headers to ensure the API's behavior is as expected.
By following these steps, you can effectively write integration tests for your Spring Boot REST API. This approach not only validates the correctness of your API but also helps catch issues early in the development cycle, leading to more robust and reliable applications.
Advanced Integration Testing Techniques (e.g., Mocking, Data Setup)
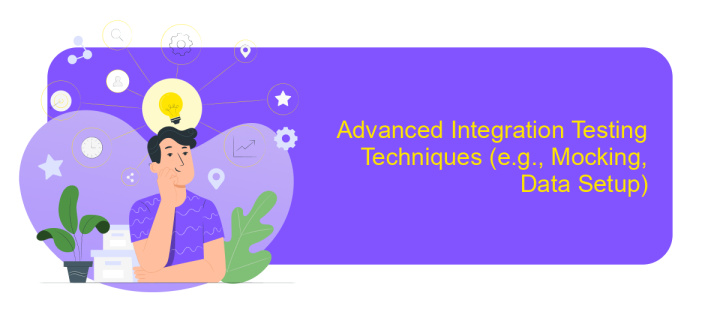
Advanced integration testing in Spring Boot involves sophisticated techniques to ensure robust and reliable REST API functionality. One such technique is mocking, which allows developers to simulate the behavior of complex, real-world services without relying on their actual implementations. This is particularly useful when external services are unstable or when testing conditions that are difficult to reproduce in a live environment.
Another crucial aspect is data setup, which involves preparing the test environment with the necessary data to execute meaningful tests. Proper data setup ensures that tests are not only repeatable but also reflect real-world scenarios accurately. This process often includes seeding databases with test data and configuring the application context to simulate different states and conditions.
- Use MockMvc for simulating HTTP requests and responses.
- Leverage @MockBean to replace specific beans in the application context.
- Utilize @Sql for setting up and tearing down database states before and after tests.
- Incorporate TestEntityManager for managing JPA entities within tests.
By employing these advanced techniques, developers can enhance their integration tests, making them more comprehensive and reflective of real-world usage. This not only improves code quality but also boosts confidence in the application's ability to handle diverse scenarios.
- Automate the work of an online store or landing
- Empower through integration
- Don't spend money on programmers and integrators
- Save time by automating routine tasks
Best Practices and Common Pitfalls
When conducting integration tests for Spring Boot REST APIs, it's crucial to maintain a clear separation between unit and integration tests. Ensure your tests are environment-independent by utilizing tools like Docker to create isolated test environments. This helps in replicating production-like scenarios without external dependencies. It's also beneficial to use Spring's @SpringBootTest annotation to load the complete application context, which facilitates comprehensive testing of your REST endpoints. Employ MockMvc to simulate HTTP requests and validate responses effectively.
Common pitfalls include neglecting the cleanup of test data, which can lead to inconsistent results. Always reset your database state between tests, possibly using tools like DbUnit. Another frequent issue is over-reliance on external services, which can be mitigated by using mocking frameworks like Mockito or integrating a service like ApiX-Drive to streamline API interactions. Lastly, ensure your tests are fast and reliable by avoiding complex setup procedures and keeping tests focused on specific functionalities.
FAQ
What is an Integration Test in Spring Boot REST API?
How do you set up an Integration Test for a Spring Boot REST API?
What tools can be used to automate Integration Testing for Spring Boot REST APIs?
How can you test different environments in Spring Boot Integration Tests?
What is the difference between Unit Tests and Integration Tests in Spring Boot?
Routine tasks take a lot of time from employees? Do they burn out, do not have enough working day for the main duties and important things? Do you understand that the only way out of this situation in modern realities is automation? Try Apix-Drive for free and make sure that the online connector in 5 minutes of setting up integration will remove a significant part of the routine from your life and free up time for you and your employees.