Integration Testing REST API in Spring Boot
Integration testing is a crucial step in ensuring the reliability and functionality of REST APIs developed with Spring Boot. By simulating real-world interactions, these tests help verify that different components of the application work seamlessly together. This article explores the best practices and tools for conducting effective integration tests in a Spring Boot environment, providing developers with the insights needed to enhance their API's performance and stability.
Introduction to Integration Testing and REST APIs
Integration testing is a crucial phase in the software development lifecycle, especially when working with REST APIs in Spring Boot applications. Unlike unit tests, which focus on individual components, integration tests evaluate the interaction between multiple components and external systems. This ensures that the different parts of an application work together seamlessly, providing a reliable and efficient user experience.
- Validates end-to-end process flows.
- Detects interface defects between modules.
- Ensures data integrity across systems.
- Simulates real-world usage scenarios.
REST APIs are a popular choice for building scalable and flexible web services. They allow different software applications to communicate over the internet using standard HTTP methods. By focusing on integration testing for REST APIs, developers can ensure that their APIs are robust, reliable, and perform as expected under various conditions. This is particularly important in Spring Boot applications, where REST APIs often serve as the backbone of microservices architecture, facilitating communication between distributed components.
Setting up the Spring Boot Test Environment
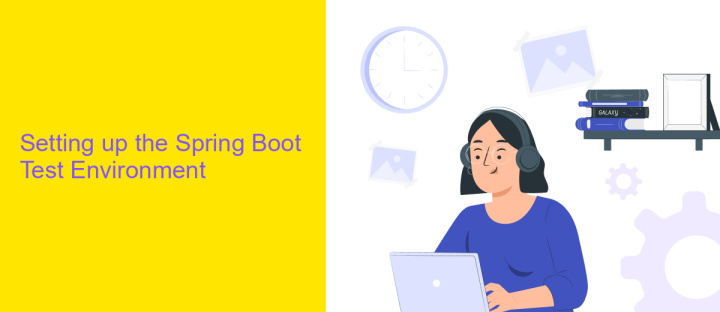
To set up the Spring Boot test environment for integration testing of your REST API, start by including the necessary dependencies in your `pom.xml` or `build.gradle` file. Ensure that you have included `spring-boot-starter-test` for testing support and `spring-boot-starter-web` for web functionalities. These dependencies provide essential libraries such as JUnit, AssertJ, and Spring Test, which are crucial for writing and executing tests effectively. Additionally, consider using Testcontainers if your application interacts with external services like databases or message brokers. Testcontainers allow you to run lightweight, disposable instances of these services during your tests, ensuring a consistent and isolated test environment.
Next, configure your application to use a test-specific configuration. This can be accomplished by creating a separate `application-test.properties` file in the `src/test/resources` directory, where you can define properties specific to the test environment. When dealing with API integrations, tools like ApiX-Drive can be beneficial for simulating and managing third-party API interactions. ApiX-Drive provides a platform for setting up, testing, and automating API workflows, which can be integrated into your test environment to ensure seamless testing of external API interactions. Finally, annotate your test classes with `@SpringBootTest` to load the complete application context and enable full integration testing capabilities.
Writing Integration Tests with MockMvc or WebTestClient
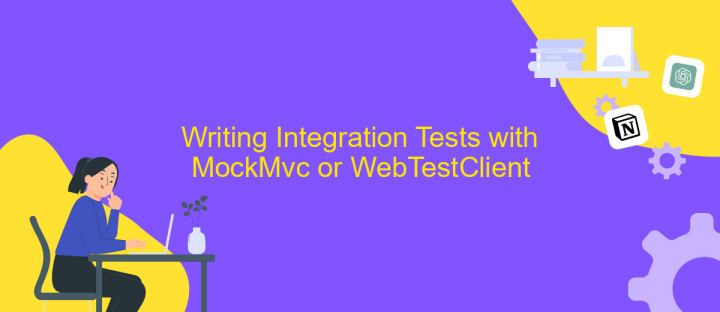
When it comes to integration testing in Spring Boot applications, MockMvc and WebTestClient are two powerful tools that can be utilized to test REST APIs effectively. Both tools allow you to simulate HTTP requests and verify the behavior of your endpoints without the need for a running server. This makes the testing process faster and more efficient.
- Set up your test class with the necessary annotations, such as
@SpringBootTest
and@AutoConfigureMockMvc
for MockMvc, or@SpringBootTest(webEnvironment = SpringBootTest.WebEnvironment.RANDOM_PORT)
for WebTestClient. - Inject either
MockMvc
orWebTestClient
into your test class. - Create test methods using
@Test
annotation, and utilize the injected MockMvc or WebTestClient to perform HTTP requests and assertions on the responses.
By leveraging MockMvc or WebTestClient, you can ensure your REST API endpoints function correctly under various scenarios. These tools offer a comprehensive approach to testing, enabling developers to catch issues early in the development cycle and maintain high-quality code standards.
Testing Different HTTP Methods and Request/Response Scenarios
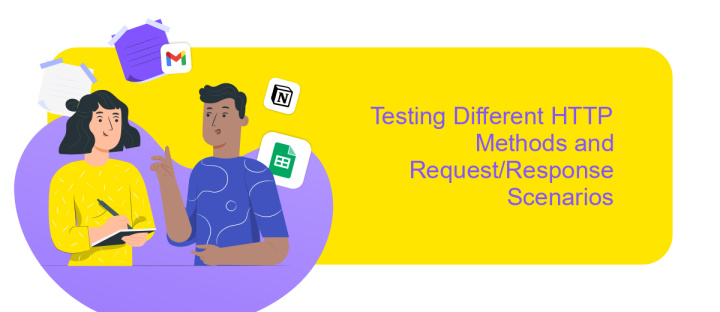
When conducting integration testing for REST APIs in Spring Boot, it's crucial to test various HTTP methods to ensure the API behaves as expected. Each HTTP method, such as GET, POST, PUT, and DELETE, serves a unique purpose and requires specific testing approaches. For instance, GET requests should be tested for correct data retrieval, while POST requests need validation for successful data creation.
Testing different request and response scenarios ensures that the API handles diverse situations effectively. This includes testing successful responses, error handling, and edge cases. By simulating various inputs and conditions, testers can verify the API's robustness and reliability.
- GET: Test data retrieval and response status codes.
- POST: Validate data creation and error responses for invalid input.
- PUT: Ensure correct data update and handling of non-existent resources.
- DELETE: Verify successful data deletion and appropriate error messages for protected resources.
By thoroughly testing each HTTP method and scenario, developers can identify potential issues early in the development process. This approach not only improves the API's quality but also enhances user satisfaction by providing a reliable and predictable service. Comprehensive testing is essential for maintaining high standards in API development.

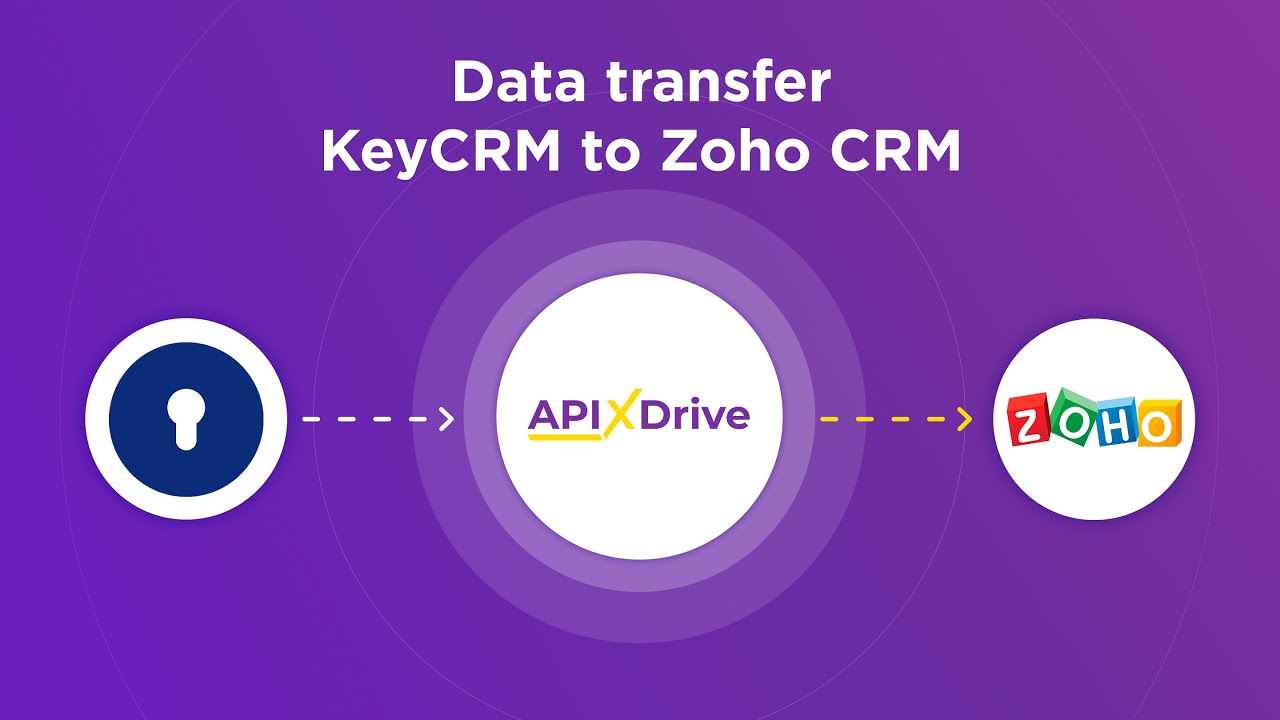
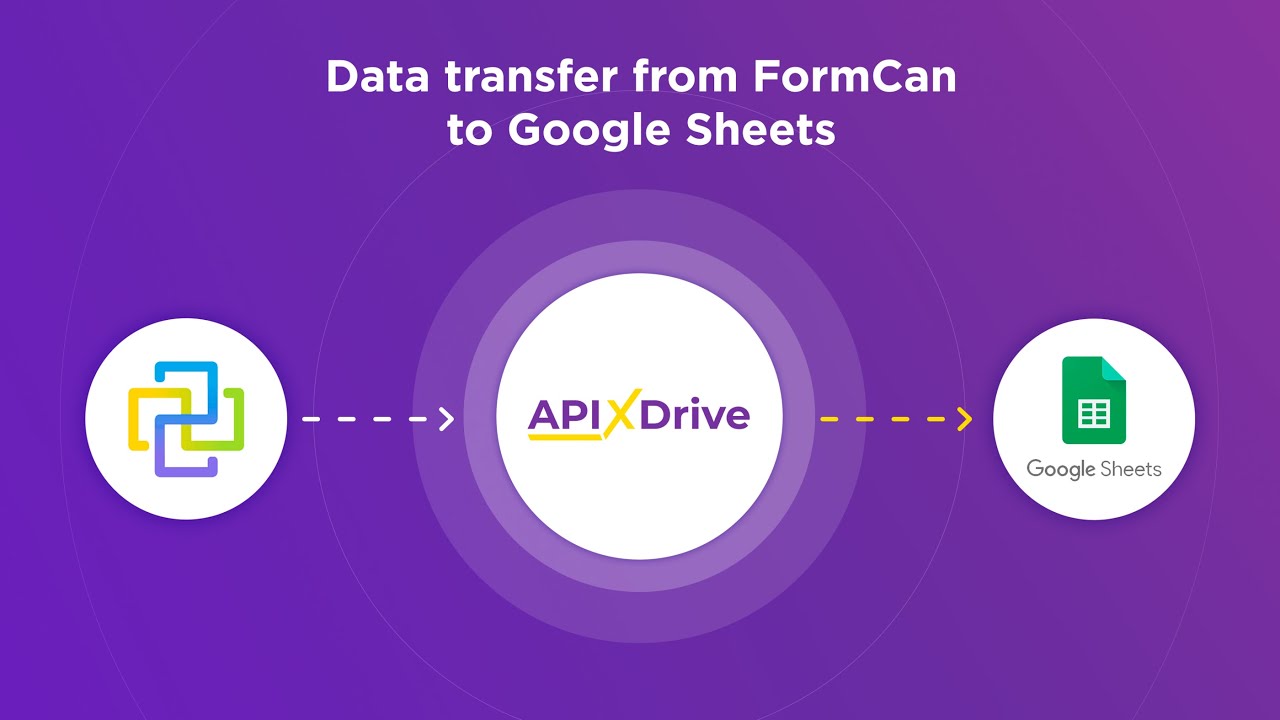
Best Practices and Common Pitfalls
When conducting integration testing for a REST API in Spring Boot, it's crucial to follow best practices to ensure efficiency and reliability. First, isolate your tests by using an in-memory database like H2 to avoid data persistence issues. Utilize Spring's @SpringBootTest annotation to load the full application context, ensuring your tests are as close to production as possible. Mock external services and dependencies to maintain test independence and speed. Additionally, consider using ApiX-Drive for seamless integration setup, which can streamline testing by automating data transfers between different applications, reducing manual errors.
Common pitfalls often include neglecting error handling and edge cases, which can lead to fragile tests. Avoid over-reliance on external APIs during testing; instead, simulate responses to ensure tests are not affected by external downtime. Moreover, failing to clean up test data can result in flaky tests due to data pollution. Ensure that each test runs in a fresh state by using @Transactional or similar mechanisms. Lastly, maintain clear and comprehensive documentation for each test case to facilitate easier maintenance and updates.
FAQ
What is Integration Testing in the context of REST API in Spring Boot?
How can I perform Integration Testing for a REST API in Spring Boot?
What are some best practices for Integration Testing REST APIs in Spring Boot?
How can I automate Integration Testing for REST APIs in Spring Boot?
What challenges might I face when Integration Testing REST APIs in Spring Boot, and how can I overcome them?
Time is the most valuable resource in today's business realities. By eliminating the routine from work processes, you will get more opportunities to implement the most daring plans and ideas. Choose – you can continue to waste time, money and nerves on inefficient solutions, or you can use ApiX-Drive, automating work processes and achieving results with minimal investment of money, effort and human resources.